C# study notes–advanced knowledge points such as data structures, generics, delegated events, etc.
C# study notes–advanced knowledge points such as data structures, generics, delegate events, etc. This blog is an advanced part of the C# study notes. It designs advanced knowledge in the C# language and introduces data structures such as List and ArrayList, Stack and Queue, and Hashtable. Generics, generic type data structures, and the tangled web of delegates and events. It also involves some uncommon but commonly used knowledge points, such as anonymous functions, Lambda expressions, covariance and contravariance, multi-threading, Knowledge points such as reflection and characteristics. Practice quietly and learn with me! C# Advanced Simple data structure class ArrayList The element type is stored in the Object type and is an array container that supports addition, deletion, and modification. Therefore, there are packing and unboxing operations, so use them with caution. //ArrayList ArrayList array=new ArrayList(); //Add ================= array.Add(“Hello”); array.Add(true); array.Add(“Tony”);//Add a single element array.Add(“Hello”); array.Add(“World”); ArrayList array2=new ArrayList(); array2.Add(“123″); array.AddRange(array2);//Add elements from the array //insert array.Insert(1,”456”); //Delete================== array.Remove(“Hello”); array.RemoveAt(1);//Remove elements based on index array.Clear();//Clear //Check================= array[0]; //Check whether the element is stored in if(array.Contains(true)) { } //Forward search for an element //Return to index. If not found, return -1. array.IndexOf(“Hello”); //Reverse search element position array.LastIndexOf(“Hello”); //Change ======================= array[2]=”123″; //Traverse====== //Distinguish…
MongoDB combines with Spring to store files (pictures, audio, etc.)
MongoDB has two ways to store images and other files (this article is aimed at users who can already use MONGODB to integrate Spring) Related reading: MongoDB backup and recovery http MongoDB has two ways to store images and other files (This article is aimed at users who can already use MONGODB to integrate Spring) Related reading: MongoDB backup and recovery CentOS compile and install MongoDB CentOS compiles and installs MongoDB and mongoDB’s php extension CentOS 6 uses yum to install MongoDB and server-side configuration Installing MongoDB2.4.3 under Ubuntu 13.04 How to create new databases and collections in MongoDB A must-read for getting started with MongoDB (paying equal attention to concepts and practice) “MongoDB: The Definitive Guide” English text version [PDF] 1.Use MongoTemplate /** * Storage file * @param collectionName collection name * @param file file * @param fileid file id * @param companyid The company id of the file * @param filename file name */ public void SaveFile(String collectionName, File file, String fileid, String companyid, String filename) { try { DB db = mongoTemplate.getDb(); // Store the root node of fs GridFS gridFS = new GridFS(db, collectionName); GridFSInputFile gfs = gridFS.createFile( file); gfs.put(“aliases”, companyid); gfs.put(“filename”, fileid); gfs.put(“contentType”, filename.substring(filename .lastIndexOf(“.”)));…
MongoDB combines with Spring to store files (pictures, audio, etc.)
MongoDB has two ways to store images and other files (this article is aimed at users who can already use MONGODB to integrate Spring) Related reading: MongoDB backup and recovery http MongoDB has two ways to store images and other files (This article is aimed at users who can already use MONGODB to integrate Spring) Related reading: MongoDB backup and recovery CentOS compile and install MongoDB CentOS compiles and installs MongoDB and mongoDB’s php extension CentOS 6 uses yum to install MongoDB and server-side configuration Installing MongoDB2.4.3 under Ubuntu 13.04 How to create new databases and collections in MongoDB A must-read for getting started with MongoDB (paying equal attention to concepts and practice) “MongoDB: The Definitive Guide” English text version [PDF] 1.Use MongoTemplate /** * Storage file * @param collectionName collection name * @param file file * @param fileid file id * @param companyid The company id of the file * @param filename file name */ public void SaveFile(String collectionName, File file, String fileid, String companyid, String filename) { try { DB db = mongoTemplate.getDb(); // Store the root node of fs GridFS gridFS = new GridFS(db, collectionName); GridFSInputFile gfs = gridFS.createFile( file); gfs.put(“aliases”, companyid); gfs.put(“filename”, fileid); gfs.put(“contentType”, filename.substring(filename .lastIndexOf(“.”)));…
Summary of usage examples of regular expressions in Go language [search, match, replace, etc.]
The examples in this article describe the usage of regular expressions in Go language. Share it with everyone for your reference, the details are as follows: Using regular expressions in Go language is very simple, sample code: The code is as follows: package test import ( “fmt” “regexp” ) func RegixBase() { //findTest() //findIndexTest() //findStringTest() //findChinesString() //findNumOrLowerLetter() FindAndReplace() } //Pass in []byte and return []byte func findTest() { str := “ab001234hah120210a880218end” reg := regexp.MustCompile(“\\d{6}”) //Six consecutive digits fmt.Println(“——Find——“) //Return the first string matching reg in str Data := reg.Find([]byte(str)) fmt.Println(string(data)) fmt.Println(“——FindAll——“) //Return all strings matching reg in str //The second parameter indicates the maximum number of results returned, passing -1 indicates returning all results DataSlice := reg.FindAll([]byte(str), -1) for _, v := range dataSlice { fmt.Println(string(v)) } } //Pass in []byte and return the first and last position index func findIndexTest() { fmt.Println(“——FindIndex——“) //Return the first and last position of the first matching string reg2 := regexp.MustCompile(“start\\d*end”) //start starts, end ends, and there are all numbers in the middle str2 := “00start123endhahastart120PSend09start10000end” //index[0] represents the starting position, index[1] represents the ending position Index := reg2.FindIndex([]byte(str2)) fmt.Println(“start:”, index[0], “,end:”, index[1], str2[index[0]:index[1]]) fmt.Println(“——FindAllIndex——“) //Return the first and last positions of…
Detailed explanation of NodeMongoose usage [Mongoose usage, Schema, objects, model documents, etc.]
The examples in this article describe the usage of Node Mongoose. Share it with everyone for your reference, the details are as follows: Introduction to Mongoose It is a framework that creates a relationship between Javascript objects and databases, Object related model. The operation object is to operate the database. The object is generated and persisted (data enters the database). Initial use of Mongoose Connect to database var mOngoose= require(‘mongoose’); //Create database connection var db = mongoose.createConnection(‘mongodb://localhost:27017/zf’); //Listen to the open event db.once(‘open’,function (callback) { console.log(‘Database connection successful’); }); module.exports = db; Define model Create schema -> scatic method defined on schema -> create model new mongoose.schema({}); //The parameter is json, defining fields. Create model db.model(collectionsName,schemaName); var mOngoose= require(‘mongoose’); var db = require(‘./db.js’); //Create a schema structure. schema–mode var StudentSchema = new mongoose.Schema({ name: {type: String, default: ‘Anonymous user’}, age: { type: Number }, sex: { type: String } }); // Create method StudentSchema.statics.zhaoren = function (name,callback) { this.model(‘Student’).find({‘name’: name},callback); } //Create modification method StudentSchema.statics.xiugai = function ( conditions,update,options,callback ) { this.model(‘Student’).update(conditions,update,options,callback); } var studentModel = db.model(‘Student’,StudentSchema); module.exports = studentModel; App.js only operates classes, not the database. var Cat = mongoose.model(‘Cat'{‘name’: String, age: Number}); Cat.find({‘name’: ‘tom’},function( err.reslut ){ var xiaomao…
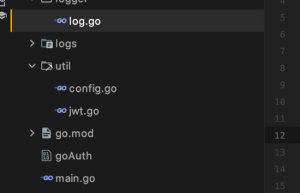
In-depth analysis of the use of golangzap log library (including file cutting, hierarchical storage and global use, etc.)
Log processing often has the following requirements: 1. Different levels of logs are output to different log files. 2. Log files are cut and stored according to file size or date to avoid a single log file being too large. 3. The log is simple and convenient to use, and can be used globally once defined. It is recommended to use Uber-go’s Zap Logger. The master Li Wenzhou’s blog has made it very clear. Please refer to Teacher Li’s blog first: https://www.liwenzhou.com/posts/Go/zap/ Questions 2 and 3 require additional description: 1. Logs are divided into files and stored according to levels 1.1 First implement two interfaces to determine the log level infoLevel := zap.LevelEnablerFunc(func(lvl zapcore.Level) bool { return lvl >= zapcore.InfoLevel }) errorLevel := zap.LevelEnablerFunc(func(lvl zapcore.Level) bool { return lvl >= zapcore.ErrorLevel }) 1.2 Get the io.Writer of info and error log files infoWriter := getWriter(“./logs/demo_info.log”) errorWriter := getWriter(“./logs/demo_error.log”) The file name can be spliced into the system time func getWriter(filename string) io.Writer { //The actual file name generated by the Logger that generates rotatelogs demo.log.YYmmddHH // demo.log is a link to the latest log // Save the log within 7 days, split the log every 1 hour (on the hour)…
Django. When I try to allow DELETE, PUT, ETC, the `actions parameter’ must be provided when calling `.as_view()`
I have to allow requests to delete and update certain model objects from the front. I wish to delete the instance and appropriate row in the database. I tried using the information from the DRF tutorial (https://www.django-rest-framework.org/tutorial/6-viewsets-and-routers/), along with some other examples. I know if using ViewSet I have to allow some operations and usage rows. I’m using decorators in the DRF tutorial. There is my view.py class DualFcaPlanUseViewSet(viewsets.ModelViewSet): authentication_classes = (CsrfExemptSessionAuthentication,) def get_queryset(self): user = self.request.user return FcaPlanUse.objects.filter(id_fca__num_of_agree__renters_id__user_key = user) def get_serializer_class(self): if self.request.method == ‘GET’: return FcaPlanUseSerializer if self.request.method == ‘POST’: return FcaPlanUsePOSTSerializer @action(detail=True, renderer_classes=[renderers.StaticHTMLRenderer]) def highlight(self, request, *args, **kwargs): fcaplanuse = self.get_object() return Response(fcaplanuse.highlighted) def perform_create(self, serializer): serializer.save(owner=self.request.user) My operations in application urls.py from django.conf.urls import url from rest_framework import renderers from .import views from cutarea.views import DualFcaPlanUseViewSet fcaplanuse_list = DualFcaPlanUseViewSet.as_view({ ‘get’: ‘list’, ‘post’: ‘create’ }) fcaplanuse_detail = DualFcaPlanUseViewSet.as_view({ ‘get’: ‘retrieve’, ‘put’: ‘update’, ‘patch’: ‘partial_update’, ‘delete’: ‘destroy’ }) fcaplanuse_highlight = DualFcaPlanUseViewSet.as_view({ ‘get’: ‘highlight’ }, renderer_classes=[renderers.StaticHTMLRenderer]) So part of my project urls.py from cutarea.views import * #… from rest_framework import routers router = routers.DefaultRouter() router.register(r’cutarea’, DualFcaPlanUseViewSet.as_view(), base_name=’cutareadel’) #… urlpatterns = [ #… url(r’^api/’, include(router.urls)), ] The result is: TypeError: The `actions` argument must be provided when calling `.as_view()`…