<Operators in Golang
Okay, I’m new to Go lang, but this doesn’t make sense to me: package main import ( “fmt” “log” ) var rectLen, rectWidth float64 = 0, 0 func init() { fmt.Println(“init is initialized”) if rectLen <0 { log.Fatal("rectLen smaller than 0") } if rectWidth <0 { log.Fatal("rectWidht smaller than 0") } } func main() { fmt.Println("Main is initialized") fmt.Println(rectLen, rectWidth) } This will print: init is initialized Main is initialized 0 0 Why are 0 and 0 printed when my init function “protects” my rectLen and rectWidth variable should be strictly greater than 0? If I change the value to something less than 0 it works fine and I get: init is initialized 2009/11/10 23:00:00 rectLen smaller than 0 Thank you! 1> Xiaozhi..: Because < is different from “equal to” .Try changing the operator to <=. This will only be triggered if your value is less than OR equal to 0
Runtime error Unable to parse all parameters of GoogleMap: (?,?)
I’m trying to load a basic map onto my template. I just followed this instruction https://ionicframework.com/docs/native/google-maps/ to get my map. But the error I get is just like the question above. This is my code // Load map only after view is initialized ngAfterViewInit() { this.loadMap(); } loadMap() { // make sure to create following structure in your view.html file // and add a height (for example 100%) to it, else the map won’t be visible // // // // create a new map by passing HTMLElement let element: HTMLElement = document.getElementById(‘map’); let map: GoogleMap = this.googleMaps.create(element); // listen to MAP_READY event // You must wait for this event to fire before adding something to the map or modifying it in anyway map.one(GoogleMapsEvent.MAP_READY).then( () => { console.log(‘Map is ready!’); // Now you can add elements to the map like the marker } ); // create LatLng object let ionic: LatLng = new LatLng(43.0741904,-89.3809802); // create CameraPosition let position: CameraPosition = { target: ionic, zoom: 18, tilt: 30 }; // move the map’s camera to position map.moveCamera(position); } } I get blank screen and I can’t print console.log in app.component.ts file Mohan Gopi.. 19 I changed my app.module.ts file import {GoogleMaps,GoogleMap}…
<>
Basic operations of channels# The channel itself is concurrency safe, and this is the only type that comes with the Go language that can satisfy concurrency safety. Channel initialization The creation of the channel is the same as the dictionary, using the built-in make function. The parameters after the make function represent the length of the chan and whether it is a buffered chan. ch1 := make(chan int, 3)//Create a chan of type int with 3 buffers Channel characteristics 1. For the same channel, sending operations are mutually exclusive, and receiving operations are also mutually exclusive. 2. The operations on elements in sending operations and receiving operations are inseparable 3. Both send and receive operations are blocked until they are completely completed. For the first feature, it means that only one user is allowed to operate when receiving and sending. Even if they are different goroutines, only one user is allowed to operate. It must be noted that when writing data into the channel, what is written is a copy of the element, not the element itself. When reading, a copy in the channel is first generated, and then prepared for the receiver, and the elements in the channel are…
Differences between some operators “|”, “^”, “&”, “&^”.Golang
Recently I read the golang specification and faced some interesting operators: & bitwise AND integers | bitwise OR integers ^ bitwise XOR integers &^ bit clear (AND NOT) integers I tried playing around with it, but the only thing I understand is that “|” adds integers and the “+” operator additionally works with floats, strings, etc. What are they used for in practice? Can someone provide some explanations for these 4 operators above? 1> icza..: When you must use byte or bit-level data, bitwise The operator comes into play. Here I list some examples of bit manipulation using code examples (in no particular order): 1.They are common in many algorithms as part of encryption and hash functions (such as MD5). 2.If you want to “save” space and you pack multiple “bool” variables into one variable, they are also often Being used with int, for example, you assign a bit to each bool variable. You have to use bitwise operators to change/read the bits individually. For example, packing 8 bits/bool into an int: flags := 0x00 // All flags are 0 flags |= 0x02 // Turn the 2nd bit to 1 (leaving rest unchanged) flags |= 0xff // Turn 8 bits…
Functions in the algorithm header file: remove() and remove_if() functions,…
remove(first, last, value) function removes the value of [first, last) range,returns the tail iterator of the new value range at the head The declaration in the file is as follows templateForwardIt remove( ForwardIt first, ForwardIt last, const T& value );//first, last are all iterators,value is a value or object,returns the last iterator of the new value range The usage method is as follows vector a = {11, 0, 2, 3, 10, 0, 0, 8, 0} ;cout <<"Original size : " < remove_if() function template ForwardIt remove_if( ForwardIt first, ForwardIt last, UnaryPredicate p ); //Remove all elements that meet specific criteria from the range [first, last) , and return Iterator after the end of the new value range. Use as follows vector a = {11, 0, 2, 3 , 10, 0, 0, 8, 0};cout <<"Original size : " <// auto itend = remove_if(a.begin(), a.end(), bind2nd(greater( ),9));//Remove numbers greater than 9 auto itend = remove_if(a.begin(), a.end(), [](int i){return i>9;});/ / You can also use lambda expressions or write a bool type function and use the function name as the third parameter. cout <<"after REMOVE, size : " < In addition,,in the header file,there are count() function and count_if() function usage and the…

java<>, >>> shift operation methods
<<, signed left shift, shifts the entire binary value of the operand to the left by a specified number of digits, and pads the low bits with 0s. int leftShift = 10; System.out.println(“Decimal:” + leftShift + “, Binary:” + Integer.toBinaryString(leftShift)); int newLeftShift = letfShift <<2; System.out.println("Shift left by 2 digits and then decimal:" + newLeftShift + ", Shift left by 2 digits and then binary" + Integer.toBinaryString(newLeftShift)); //The decimal result of positive integer x after shifting n digits to the left, x = x * 2^n The above are positive integers, and the operation results are as follows. Next, let’s take a look at what happens when a negative number is left shifted by 2 bits. The result of the operation is as follows. Why does the binary number -10 have so many 1’s? If you count carefully, there are exactly 32 bits. The first thing you need to understand is that Java negative numbers are stored in two’s complement form (complement = complement + 1). The binary number of 10 is 1010, and its complement is 0101. Adding 1 is the complement 0110. So why are there so many extra 1’s? This is because the int type occupies 8…
Detailed introduction to the difference between javaequals and =, ==
The difference between equals and == in Java Data types in Java can be divided into two categories: 1. Basic data types, also called primitive data types. byte, short, char, int, long, float, double, boolean To compare them, use the double equal sign (==), and compare their values. 2. Composite data type (class) When they use (==) to compare, they compare their storage addresses in memory. Therefore, unless they are the same new objects, their comparison results are true, otherwise the comparison results is false. All classes in JAVA inherit from the base class Object. An equals method is defined in the base class in Object. The initial behavior of this method is to compare the memory addresses of objects, but in some class libraries This method has been overridden. For example, String, Integer, and Date have their own implementations of equals in these classes, instead of comparing the storage address of the class in the heap memory. For equals comparison between composite data types, without overriding the equals method, the comparison between them is still based on the address value of their storage location in memory, because the equals method of Object also uses double The equal sign (==)…
JavaScript operators (~, &, |, ^, <>) use cases
Stop talking nonsense and get straight to the code The code is as follows: The explanation is very clear in the comments. Do you guys understand the usage of operators in Javascript? If you have any questions, please leave me a message
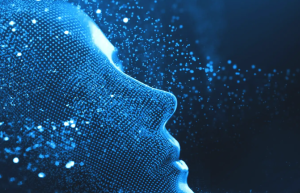
<<<java environment setup
First search “jdk download” on Baidu After the installation is complete, go to the system environment variable settings (right-click on the computer, properties, advanced system settings) Then confirm, OK Go to CMD to test it Method: Run cmd and enter java respectively -version and javac – version <<<java environment setup, bubuko, bubuko.com <<<java environment setup
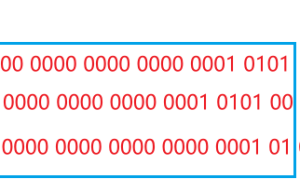
[Java Basics] Bit operations <>, >>>, &, |, ^, ~
1. Bit operator Bit operators perform operations on the binary system of integers. 2. Bit operator details <<: Within a certain range, each shift to the left by 1 bit is equivalent to * 2 >>: Within a certain range, each shift to the right by 1 bit is equivalent to / 2 >>> represents an unsigned right shift operation symbol. Filling the high bit with 0 For example, 8>>>2 means shifting 8 to the right by 2 bits, and the result is 2. This can also be understood together with the right shift operator. 3. Example For positive numbers, fill the highest vacated bit with 0 For negative numbers: >>After the right shift, the highest vacated bit is filled with 1 >>> After the right shift, the highest vacated bit is filled with 0