
In Java, when creating a subclass object, will the parent class object be created first? (What if the parent class is an abstract class?)
https://blog.csdn.net/banzhengyu/article/details/81039757 In Java, when creating a subclass object, will the parent class object be created first? (What if the parent class is an abstract class?) Ask a question: If when creating a subclass object, the parent class object will be created first, then if the parent class is an abstract class, then the abstract parent class will also be instantiated. This is different from the abstract class. Can’t instantiate each other! package javase5; public abstract class Animal { int age; public Animal() { this.age = 6; System.out.println(“I am the parameterless constructor of the Animal class”); } } package javase5; public class Cat extends Animal{ public Cat() { // super(); //Even if you don’t write the super(); line of code, the system will automatically call the parent class’s no-argument constructor by default. System.out.println(“I am the parameterless constructor of Cat class”); } public static void main(String[] args) { Cat cat = new Cat(); System.out.println(cat.age); Cat cat2 = new Cat(); System.out.println(cat2.age); } }
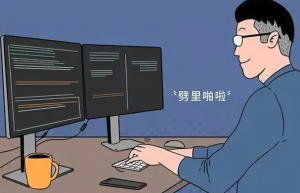
In java, read the data in the file item by item according to the delimiter in the file (space, newline, etc.)
In Java, the data in the file is read one by one according to the delimiters (spaces, newlines, etc.) in the file In C++, the data in the file can be read sequentially according to the delimiters such as spaces, newlines, tabs, etc. in the file To get the data in the file, I recently encountered a similar problem in the java project. In java, I also need to read all the data in the file based on the delimiter. I searched for the relevant javadoc documents and found a useful class called Scanner. Next, describe the use of this class in this problem: Scanner’s description in javadoc The scanner can also use delimiters other than whitespace. This example reads several items in from a string: String input = “1 fish 2 fish red fish blue fish”; Scanner s = new Scanner(input).useDelimiter(“\\s*fish\\s*”); System.out.println(s.nextInt()); System.out.println(s.nextInt ()); System.out.println(s.next()); System.out.println(s.next()); s.close(); prints the following output: 1 2 red blue import java.io.File;import java.io.FileNotFoundException;import java.util.Scanner;public class Test { public static void main(String[] args) throws FileNotFoundException { File file = new File(“./src/base/network/test.txt”); if(!file.exists()) { System.out.println(“Node basic information file not found”); System.exit(0); } Scanner s = new Scanner(file); String nodeNum = s .next(); int num = s.nextInt();…
What is the difference between &, |, &&, || in Java
This article will introduce to you the differences between &, |, &&, and || in Java. It has certain reference value. Friends in need can refer to it. I hope it will be helpful to everyone. int i=0; if(3>2 || (i++)>1) i=i+1; System.out.println(i); This program will print out 1 instead of 2. Because in the conditional judgment of if, the program first judges whether the first expression 3>2 is true, and the result 3>2 is true, then according to logic, regardless of the following expression (i++) >1 is true, the entire OR expression must be true, so the program will not execute the judgment of the next expression, that is, (i++)>1, so i does not increase by 1 here. Then the program executes until i=i+1, so i becomes 1. Finally print out 1. int i=0; if(3>2 | (i++)>1) i=i+1; System.out.println(i); If you write it like this, it will print out 2, because no matter whether the first condition 3>2 is true or not, the program will execute the judgment of the second condition Conditional expression, so the increment of i++ will be executed, plus i=i+1 in if, so eventually i=2. Some people are used to calling || a short-circuit or,…
In java, its calling method is implemented by class name and method name string
In js, through the eval() function, you can call a method after knowing the name of the method. So how is it implemented in java? Java is implemented through the reflection mechanism. The code is as follows: import java.lang.reflect.Method; public class Test { public static void main(String[] args) throws Exception { String className = “com.runqianapp.ngr.alias.example.FunClass”; String methodName = “sayHello”; Class clz = Class.forName(className); // Object obj = clz.newInstance(); //get method Method m = obj.getClass().getDeclaredMethod(methodName, String.class); //Call method String result = (String) m.invoke(obj, “aaaaa”); System.out.println(result); } } class FunClass{ public String sayHello(String s){ System.out.println(s); return “hello!”; } } Supplementary knowledge:A controller call distributes different services according to different businesses In a project, you need to write many controllers to call different services, and writing a gateway can save you the pain of writing the controller layer. The following begins with an introduction to the different services that can be distributed. 1. Because the service has all been injected into the spring container when the project is started, we need to write a tool class that can obtain the corresponding service from the spring context (applicationContext) @Component public class SpringUtil implements ApplicationContextAware { @Autowired private static ApplicationContext applicationContext; @Override public void setApplicationContext(ApplicationContext…
In java, what is responsible for interpreting and executing byte code?
In java, what is responsible for interpreting and executing byte codes? In Java, the virtual machine is responsible for interpreting and executing byte codes. The execution mode of the Java language is semi-compiled and semi-interpreted. Programs written in Java are first converted into standard byte codes by the compiler, and then interpreted and executed by the Java virtual machine. Byte code is a binary file, but it cannot be run directly on the operating system. It can be regarded as the machine code of a virtual machine. The virtual machine separates the bytecode program from each operating system and hardware, making the Java program independent of the platform. The virtual machine in Java is a very important concept and is the basis of the Java language. Mastering it will help you understand the implementation of the Java language. Recommended tutorial: “Java Learning” The above is the detailed content of what is responsible for interpreting and executing byte codes in Java. Please pay attention to other related articles for more!

In Java, s.charAt(index) is used to extract specific characters in string s.
The charAt(int index) method is a method that can be used to retrieve a String instance of a character at a specific index. The charAt() method returns the char value at the specified index position. The index range is 0~length()-1. For example: str.charAt(0) retrieves the first character in str, str.charAt(str.length()-1) retrieves the last character. Warning: Accessing an out-of-bounds character in a string s is a common programming error. To avoid such errors, make sure that the subscript used does not exceed s.length()-1. public class hash { public static void main(String[] args) { // TODO Auto-generated method stub String str = “This is yiibai”; // prints character at 1st location System.out.println(str.charAt(0)); // prints character at 5th location i.e white-space character System.out.println(str.charAt(4)); // prints character at 18th location System.out.println(str.charAt(6)); } } The results are as follows: Supplementary knowledge:Two commonly used methods for extracting strings in JAVA (subString, regular expressions) 1. Operate strings through the subString() method subString() syntax: public String substring(int beginIndex) or public String substring(int beginIndex, int endIndex) 1. The obtained string length is: endIndex – beginIndex; 2. Start from beginIndex and end at endIndex, counting from 0, excluding the characters at endIndex Example: String str1=”abdc686512ffsc1267d”; String substr1=str1.substring(4); //Specify the interval…
In java, why is it not important that we use classes in code before declaring it?
In Java, it is common to write main methods above (or at least I do), and in these methods, objects are created even if their respective classes are declared below. Why doesn’t this cause a compilation error? I know java is not procedural, but I never found the answer. public static void main (String[] args) { baby1 obj1 = new baby1(); baby2 obj2 = new baby2(); } class baby1 { //some code } class baby2 { //some code } Xiao Zhi.. 6 Java uses multiple passes for name resolution. The first pass is the “definition pass”, which scans the source program and stores classes,fields in tables > and Method. In the next pass (“parse pass”), if a class is encountered, it will look for that class in the table created in the previous pass. If The class is there, it will continue, otherwise an error will be thrown. However, this does not happen with local variable declaration. You cannot use local variables before declaration. 1> Xiaozhi..: Java uses multiple passes for name resolution. The first pass is the “definition pass”, which scans the source program and stores classes,fields in tables and Method. In the next pass (“parse pass”), if a…
In java, why doesn’t stream peek work for me?
1> davidxxx..: Because peek() accepts Consumer. A Consumer is designed to accept parameters but return no results. For example here: genre.stream().peek(s-> s.replace(“r”,”x”)) s.replace(“r”,”x”) is indeed executed, but it does not change the content of the stream. It is just a method-scoped String instance that goes out of scope after calling peek(). To test, replace peek() with map(): List genre = new ArrayList(Arrays.asList(“rock”, “pop”, “jazz”, “reggae”)); System.out.println(genre.stream().map(s -> s.replace(“r”, “x”)) .peek(s -> System.out.println(s)) .filter(s -> s.indexOf(“x”) == 0).count());
In Java, which thread executes sequential flow?
java foreach stream Write your review! Come on, watch it all Member login | User registration Recommended reading stream Common methods for Java IO reading and writing Java’s streams are divided into two categories, byte streams and character streams. Generally in cc++, one byte is 8 bits, and the same is true for java. However, in cc++, a character, that is, char, is generally 8 bits (may vary depending on the machine), but in java… [detailed] Crayon Shin-chan 2023-10-17 20:31:55 web linux resin log, linux resin basic site configuration Enter the configuration file directory: [rootlinuxidcresin-4.0.]#cdusrlocalresinconf to see what configuration files there are: [rootlinuxid… [detailed] Crayon Shin-chan 2023-10-17 17:36:18
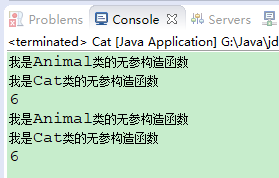
In Java, when creating a subclass object, will the parent class object be created first? (What if the parent class is an abstract class?)
https://blog.csdn.net/banzhengyu/article/details/81039757 In Java, when creating a subclass object, will the parent class object be created first? (What if the parent class is an abstract class?) Ask a question: If when creating a subclass object, the parent class object will be created first, then if the parent class is an abstract class, then the abstract parent class will also be instantiated. This is different from the abstract class. Can’t instantiate each other! package javase5; public abstract class Animal { int age; public Animal() { this.age = 6; System.out.println(“I am the parameterless constructor of the Animal class”); } } package javase5; public class Cat extends Animal{ public Cat() { // super(); //Even if you don’t write the super(); line of code, the system will automatically call the parent class’s no-argument constructor by default. System.out.println(“I am the parameterless constructor of Cat class”); } public static void main(String[] args) { Cat cat = new Cat(); System.out.println(cat.age); Cat cat2 = new Cat(); System.out.println(cat2.age); } }